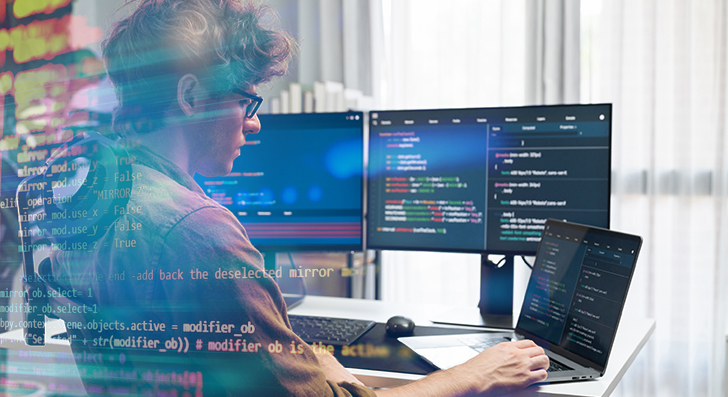
Scalability usually means your application can handle advancement—additional consumers, much more details, plus more website traffic—with no breaking. Like a developer, building with scalability in your mind saves time and worry later on. Right here’s a transparent and useful tutorial that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part of your system from the beginning. A lot of applications fall short when they increase fast mainly because the original design can’t take care of the additional load. As being a developer, you might want to Believe early regarding how your system will behave under pressure.
Start out by planning your architecture to be versatile. Stay clear of monolithic codebases exactly where anything is tightly related. As an alternative, use modular style and design or microservices. These styles break your application into smaller, impartial areas. Each module or services can scale on its own devoid of influencing the whole program.
Also, think about your database from day one particular. Will it have to have to handle a million consumers or maybe 100? Pick the proper variety—relational or NoSQL—based upon how your details will mature. Approach for sharding, indexing, and backups early, even if you don’t want them still.
Another vital point is to prevent hardcoding assumptions. Don’t compose code that only performs underneath latest conditions. Consider what would occur Should your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use design patterns that assistance scaling, like concept queues or function-driven devices. These assistance your application tackle extra requests without the need of having overloaded.
After you Establish with scalability in your mind, you are not just preparing for fulfillment—you might be minimizing foreseeable future complications. A very well-planned technique is simpler to keep up, adapt, and grow. It’s better to arrange early than to rebuild later on.
Use the correct Database
Deciding on the suitable database is really a important A part of creating scalable applications. Not all databases are built a similar, and utilizing the Improper one can gradual you down as well as result in failures as your application grows.
Begin by understanding your details. Could it be very structured, like rows inside a desk? If Indeed, a relational database like PostgreSQL or MySQL is an effective fit. These are definitely strong with interactions, transactions, and consistency. In addition they assist scaling methods like examine replicas, indexing, and partitioning to deal with extra targeted traffic and info.
If your information is much more flexible—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at managing large volumes of unstructured or semi-structured data and may scale horizontally additional effortlessly.
Also, look at your read and publish styles. Have you been executing plenty of reads with less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases that could handle large produce throughput, or even occasion-centered info storage units like Apache Kafka (for temporary info streams).
It’s also clever to Imagine ahead. You may not require Superior scaling capabilities now, but deciding on a database that supports them implies you gained’t need to have to change afterwards.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data based on your accessibility patterns. And generally observe databases general performance when you mature.
To put it briefly, the right databases relies on your application’s composition, pace needs, and how you hope it to mature. Choose time to select correctly—it’ll preserve plenty of problems later.
Optimize Code and Queries
Speedy code is key to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your method. That’s why it’s crucial to build economical logic from the beginning.
Start off by composing thoroughly clean, simple code. Stay clear of repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a straightforward a person performs. Keep your capabilities limited, focused, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take also extended to operate or employs too much memory.
Upcoming, examine your databases queries. These usually gradual items down more than the code by itself. Make sure Every single query only asks for the info you actually need to have. Steer clear of Pick out *, which fetches every thing, and as a substitute choose distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, In particular across substantial tables.
In the event you observe a similar information staying asked for repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached and that means you don’t really have to repeat costly functions.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your app much more productive.
Make sure to take a look at with huge datasets. Code and queries that operate high-quality with a hundred records may crash after they have to manage one million.
Briefly, scalable applications are rapid applications. Keep the code tight, your queries lean, and use caching when essential. These techniques enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers and more visitors. If almost everything goes by way of one particular server, it is going to promptly turn into a bottleneck. That’s the place load balancing and caching are available in. These two tools help keep your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic throughout a number of servers. As an alternative to a single server carrying out all of the function, the load balancer routes users to distinctive servers based upon availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing data quickly so it may be reused rapidly. When buyers request exactly the same information and facts yet again—like a product web site or simply a profile—you don’t ought to fetch it from your databases whenever. You are able to provide it through the cache.
There are two prevalent kinds of caching:
one. Server-side caching (like Redis or Memcached) suppliers info in memory for fast entry.
2. Shopper-side caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, increases speed, and can make your app far more efficient.
Use caching for things that don’t improve usually. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application handle a lot more buyers, stay rapidly, and Get better from troubles. If you propose to grow, you may need both of those.
Use Cloud and Container Tools
To create scalable apps, you would like resources that allow your app improve easily. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to rent servers and companies as you require them. You don’t really have to buy hardware or guess future capacity. When visitors raises, it is possible to incorporate more resources with just a few clicks or automatically using auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can focus on building your application in place of taking care of infrastructure.
Containers are A different vital Software. A container deals your app and every thing it needs to operate—code, libraries, options—into one particular unit. This can make it uncomplicated to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Software for this.
Whenever your app uses various containers, equipment like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it automatically.
Containers also enable it to be simple to separate portions of your app into expert services. You'll be able to update or scale parts independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you'd like your application to develop devoid of limits, start off using these instruments early. They save time, lessen risk, and allow you to continue to be focused on creating, not correcting.
Keep track of Almost everything
For those who don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make superior conclusions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking standard metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—check your app way too. Regulate how much time it takes for customers to load pages, how often errors occur, and in which they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential difficulties. As an example, Should your response time goes above a Restrict or simply a company goes down, you'll want to get notified straight away. This can help you fix challenges speedy, generally in advance of end users even recognize.
Monitoring is also practical more info any time you make alterations. Should you deploy a brand new feature and find out a spike in mistakes or slowdowns, you are able to roll it again ahead of it triggers genuine destruction.
As your application grows, visitors and data raise. Without having monitoring, you’ll miss indications of difficulty right until it’s way too late. But with the correct applications in position, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works properly, even stressed.
Ultimate Views
Scalability isn’t just for significant organizations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Construct applications that develop efficiently without breaking under pressure. Start out small, Consider significant, and Construct clever.